ASP.NETSelectively Enable Form Validation When Using ASP.NET Web Controls
James M. Venglarik, IIThis article assumes you‘re familiar with ASP.NET and Visual Basic .NETLevel of Difficulty 1 2 3 Download the code for this article: Valid.exe (38KB)SUMMARY Sometimes the extra controls thatcome with Visual Studio .NET can be a bit inflexible or they just don‘tprovide enough functionality or flexibility for all situations. TheASP.NET form validation controls, while powerful and easy to use,require that the entire page be valid before it‘s submitted back to theserver. Through the use of the new object-oriented features of VisualBasic .NET, it is possible to extend their functionality to overcomethis limitation.
Thisarticle tells you how and helps you decide when it‘s a good idea tokeep validation on the client and when you‘d be better off disablingit.
nASP.NET, you can include validation controls that automatically checkuser input on a page. While this is not a new function (it wasavailable through JavaScript include files), the implementationcertainly is. You no longer have to worry about where to do validation(on the server or client), nor do you have to worry about theclient-side code. It‘s all provided by means of using the five built-invalidation controls: Regular Expression, Required Field, Range,Comparison, and Custom.
Muchhas already been written about the use of the validation controls, so Iwon‘t go into the functionality of each. For those details, see AnthonyMoore‘s articles "
User Input Validation in ASP.NET" and "
ASP.NET Validation in Depth".Suffice it to say that it‘s fairly easy to include the controls on yourpage; simply specify any parameters that are needed (the control tovalidate, the regular expression to validate against, and so on), andlet it run. That is the beauty of the new controls. You don‘t have toworry about how they are implemented, just that they are there andfunctioning.
However,by including those validation controls on your page, you are implyingthat you want all of the user‘s input validated before acting upon it.Even if this isn‘t the intended effect, you might find out that it iseasiest to include these controls to check all the input. In thisarticle, I will explain why you might want to disable those controlsand how to do so. I will show you how to disable controls for an entirepage, how to disable them from the server, and how to disable them incertain instances on both the client and the server.
The Validation ProcessThe following is a simplified description of the process that happens when a validation control is used on a page.
- When a page containing validation controls is requested, the user‘s browser type and version is evaluated to determine if client-side validation routines should be used.
- If the browser supports client-side validation, the page loads with the appropriate routines being called from the validation controls. This is handled through a JScript® include file.
- When the user moves between controls on a client-side validated form after changing a control‘s value, the validation events for the control that lost the focus are fired and appropriate error messages (if any) are displayed.
- If the user generates a form submit on a client-side validated form, the entire form is evaluated for any validation controls that are not valid. If even one control is not valid, the form will not be submitted.
- When the form is posted back to the server through a postback event, it is evaluated for validity on the server. This occurs even if the form was already evaluated on the client.
- If any of the validation controls are found to be invalid through the server-side check, the page is redisplayed with the appropriate error messages included.
- If the form passes all validation on both the server and client, the page processing sequence continues with the event that triggered the postback firing.
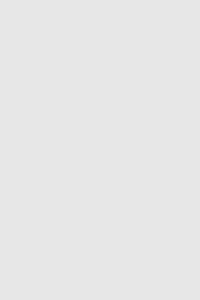
Asyou can see, the validation can occur multiple times on the client aswell as on the server. This provides enough validation so that ifimplemented properly, almost no erroneous data will be passed. Attimes, however, you might want to circumvent this process. Let‘s seewhy.
Reasons to Disable Form ValidationWhileform validation is a great tool for making sure that invalid orincorrectly formatted data is never acted upon, there are certainsituations in which you might want the data to pass through. Oneinstance, as Anthony Moore‘s ASP+ Validation article suggests, is whenyou leave the form by means of a Cancel button. Frequently, whenentering an order, updating an FAQ, or registering, the user will wantto back out of what they are doing. You can provide a link on the pagethat navigates elsewhere, thereby discarding all of the entered data,but for consistent design every Submit button should have a matchingCancel button.
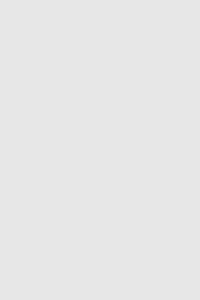
Anotherexample is a multipart form. Since you can only have one server-sideform (runat=server), you cannot divide the page into multiple formsthat will generate postback events. If you have a page that lists FAQsat the top for editing, but at the bottom of the page you provide themeans to add a new FAQ, you do not want the errors in editing toprevent someone from adding a new FAQ to the page.
The trick in these instances is to know when to allow validation and when to prevent it.
Disabling Client-Side Validation on the Entire FormAneasy way to disable all client-side form validation is to provide theclienttarget=downlevel attribute in the Page directive at the top ofyour page. The directive looks like this:
<%@ Page Language="VB" codebehind="MyPage1.vb" InheritsMyApp.MyPage1" clienttarget=downlevel>
You need to be careful when you do this because it not only disablesall client-side validation, it also disables any other client-sideserver control functionality. You can also disable client-side formvalidation for all validation controls by setting the global pagevariable Page_PostIfValidationError to True.
Theonly time you would do either of these is when you have included somevalidation controls through a common include for the page or embeddedthem in a custom control. Alternately, you can opt not to include thevalidation controls on the page.
Youcan disable all client-side form validation using a number of differentmethods depending upon the type of control that the user activates. Fora regular HTML control, you can set the Page_ValidationActive propertyto false. For example:
<input type=button runat=server id=cmdCancel value="Cancel"onClick="Page_ValidationActive=false;"onServerClick="cmdCancel_Click">
Please note that it is important to set the onServerClick event toequal the event you have defined in the codebehind page for thecontrol; otherwise, the event won‘t fire since you have overridden theonClick event.
For a server-side control, disabling all form validation requires some client-side code, like this:
<asp:button runat=server id=cmdCancel Text="Cancel"onClick="cmdCancel_Click"><script language="JavaScript>document.all["cmdCancel"].onclick =new function("Page_ValidationActive=false;");</script>
The effect of these last two examples is that all client-sidevalidation has been turned off when the Cancel button is pressed. Youwill still have to bypass server-side validation if the server codeuses the Page.IsValid property.
Thereare a few drawbacks with these examples that I‘d like to mention.First, you will receive a JavaScript error on the client when executingthe code in the examples in any browser other than Microsoft® InternetExplorer 4.0 or higher. The reason is that the Page_ValidationActiveproperty only exists when the user is using Internet Explorer 4.0 orhigher. Also, the examples turn off validation for the entire form, notjust certain controls. I will show you how to get around thesedrawbacks.
Disabling Server ValidationAnotherway to disable form validation is through server-side programming.There are a variety of ways to accomplish this task through thecontrol‘s Enabled, Visible, and Display properties. Each has its prosand cons. You should be careful to note which properties disablevalidation on the client versus the server and be aware of the effectthis might have on overall validation.
Disablingvalidation through server-side code gives you the flexibility to turnoff validation for certain controls and not the entire page, and alsoallows this to be accomplished at run time. This is important since youcan respond to certain events before you decide to turn off thevalidation. For example, based on a user‘s security level, you mightwant a field to be required only at specific times.
Disabling Client ValidationAsI mentioned, there are drawbacks to disabling all client-side formvalidation with the methods already discussed. You can circumvent thesedrawbacks by using code I‘ll describe in this section.
Ifyou‘re like me and want to separate display code from processing code(even to go as far as not embedding JScript in the .aspx file), you cancreate your own custom control for turning off client-side andserver-side validation for certain validation controls. I will gothrough a simple example of how to disable multiple validation controlsfrom a server-side button control without putting any JScript functionsin the .aspx file.
Ibuilt a small application, MyApp, that has one page (MyPage1). The pageincludes a field for editing the number of copies of a book ininventory (I‘ll assume the user has chosen this on another page). Thereis a button to cancel the editing (which acts as a reset button) andone to update the number of copies.
Figure 1 Valid NumberYou can also add a new title to the inventory along with the number of copies that are available.
Figure 1 shows the page when it is first displayed.
Figure 2shows the page when a user has provided invalid input in the Editsection‘s Number Available field and moved the focus to anothercontrol. Placing the cursor over the error image displays the error anda solution.
Figure 2 Invalid EntryIncludedin the page are form validation controls for each of the fields. Allfields have a Required Field Validation control, while the numericfields have a Regular Expression Validation control to make sure theinput value is numeric. When the user clicks Save Changes, I want theform to be passed back even though there may be errors in the Addsection of the form. The same is true when the user clicks Add toInventory when there may be errors in the Edit section of the form.
Building the Custom ControlFigure 3shows all the code for this custom control, which takes the place of aregular Submit button and turns off client-side validation. Let‘s walkthrough the code step by step.
Thefirst detail to notice is the definition of the namespace. Whencreating a Web Control through the Visual Studio® .NET IDE, this isn‘tautomatically included. I included it in order to reference the controlon my Web page.
Next,I made sure the custom control extended the functionality of a basecontrol. In this instance, I used a button control as the base for thecustom control. I extended this functionality with the line:
Inherits System.Web.UI.WebControls.Button
Thenext step was to set up a property to accept a list of form validationcontrols that I wanted the custom control to disable. I accomplishedthis by setting up a public property that I called NoFormValList anddeclared the Get and Set procedures, which store their value in theState object for persistence and easy retrieval.
One important thing to note about the Get procedure is the use of the statement:
If o Is Nothing Then
It took me a while to figure out what happened to our old friend Null.Since everything is now an object, Null in Visual Basic® .NET isreplaced with Nothing. The only exception to this occurs when returninga value from the database; in this case the value can be of typeSystem.DBNull. So now, instead of using the IsNull function, everythingshould be tested against Nothing. Also note that when testing againstobjects, you need to use the Is operator.
Next,I needed to override the Render method of the button control because Iwanted to make it emit extra code if the browser allowed client-sidevalidation. Within this subroutine, the first thing I needed to check,after making sure there were validation controls to disable, was whichbrowser was being used. The following line obtains a reference to theRequest object on the page:
Dim theRequest As System.Web.HttpRequest = _System.Web.HttpContext.Current.Request
Once I had this reference, I checked against it for the browser typeand major version. If the browser is Internet Explorer 4.0 or higher, Iknow that client-side validation will occur. (For more information onthe HttpContext object, check out
The ASP Column in the July 2001 issue of
MSDN®
Magazine.)
Withinthis conditional, I used the Output object to send text to the HTMLpage. I began by setting up a JScript function that does the actualdisabling of the specified validation controls. I appended thecontrol‘s ID property to the name of the function so that the functionwas uniquely identified on the page. If I didn‘t do this but leftmultiple instances of this control on the page, all of the functionswould be named exactly the same and only the last one declared would beexecuted.
Then,using the Split function to put the list into an array, I loopedthrough the array generating the JScript code necessary to disable thevalidation control. The client-side ValidatorEnable function handledthis for me. By specifying false, the function disabled the validationcontrol.
Oneimportant thing to note about the loop itself is the use of the newGetUpperBound method. This method is available in arrays and, unlikethe Visual Basic 6.0 UBound function, it actually returns the upperbound of the array. For instance, if I had a zero-based,single-dimension array of 10 items, it would contain elements 0 through9. The Visual Basic 6.0 UBound function would return 10, but the newGetUpperBound method will return 9. Also note that the GetUpperBoundmethod requires the dimension for the upper bound. This is alsozero-based, so the first dimension is referenced as 0.
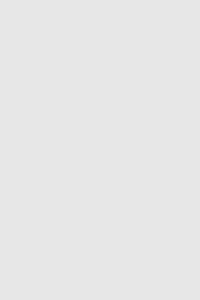
AfterI closed out the function, I added a couple of attributes to thecontrol‘s output. The first was the onServerClick attribute, whichtells the page which method to execute when it returns to the server. Ineeded to set this, otherwise when I appended the normal onClickattribute the application wouldn‘t know to execute anything on theserver side. I constructed the attribute‘s value based on the control‘sID. This gave me the default name of the event on the server.
Thesecond attribute I appended was the onClick attribute. This overridesthe normal onClick event and points its value to the new JScriptfunction I just defined. When ASP.NET renders the page, it adds anonClick attribute to any Submit buttons for client-side validationpurposes. Since I handled the validation manually, I included the codethat ASP.NET would have inserted into the extra onClick attribute inthe onClick attribute I added. This extra code is what calls theclient-side validation routines and is specified as:
if typeof(Page_ClientValidate) == ‘function‘)Page_ClientValidate();
Later I will show you how to disable the extra onClick attribute from appearing.
Nowwhen the user clicks on the button, the client-side onClick functionexecutes. When it gets to the server, the normal server-side
ControlName_Click event fires.
Thelast two lines of the routine apply to all browsers—not just InternetExplorer 4.0 or higher. The first line prevents ASP.NET from insertingthe client-side validation call into an extra onClick attribute. Thelast line of the routine, MyBase.Render(output), writes out the codefor the control. Notice that it is outside of the conditional since Ihave to render the control no matter what browser is being used.
NextI needed a way to turn off the server-side validation of the samevalidation controls. The DisableServerSideValidation method handlesthis for me—I just needed to call it in the right place.
Thefirst thing this method does, after checking to make sure there arevalidation controls to disable, is break up the list of validationcontrols that you want to disable into an array. The code loops throughthe array again, but this time for server-side processing. I used theFindControl function to obtain a reference to the current control inthe list.
Aftervalidating that the control exists (note the use of the Is operatoragain), I used a Select Case conditional on its type to determine whatto do next. In each of the Case statements, I converted the controlinto its real type and set its IsValid property to True. Since all ofthis occurs after all of the validation controls have been validated,this has the effect of overriding the invalidity of any of thevalidation controls in the list. Therefore, when the Page.IsValidproperty is called, it will return True (as long as the othervalidation controls not in the list are valid).
That‘sit for the custom control. Let‘s move on to the page itself and see howto use this new control to turn off the validation.
Using the Custom Control in the DisplayThedisplay portion of the page is in the .aspx file. At the top of thepage, just under the Page declaration, I included a reference to thenew control‘s namespace so that I could use the control on the page. Idid this by making the following declaration:
<%@ Register TagPrefix="nfvc"Namespace="MyApp.NoFormValControls" Assembly="MyApp" %>
Note the use of the application name in the namespace attribute. I haveseen many instances where the application name is not used, but I havenot gotten the registration to work without it. Also note that theAssembly attribute is required. I simply set it to the name of theapplication.
NowI can put my control within the server-side form. You can get the fullcode for the MyPage1.aspx file at the link at the top of this article.Next, I‘ll look at adding the Save Changes button to the page.
Let‘sgo ahead and assume that the Add section‘s Title field has a requiredfield validator with the ID of valReqTitle and that the Add section‘sNumber Available field has a regular expression validator and arequired field validator with the IDs of valREAdd and valReqAdd,respectively. I then put the Save Changes button on the form with thefollowing code:
<nfvc:NoFormValButton id="Save"runat=Server text="Save Changes >>"NoFormValList="valReqTitle,valREAdd,valReqAdd"></nfvc:NoFormValButton>
Using the TagPrefix of nfvc that I defined at the top of the page, Ideclared the NoFormValButton control, setting the runat, id, and textattributes. I also set the new NoFormValList attribute to acomma-separated list of validation controls I want to turn off when theSave Changes button is clicked.
Figure 4shows the source code when viewed through the browser for InternetExplorer 4.0 or higher. For all other browsers (that don‘t support theclient-side validation), the control looks like the following:
<input type="submit" name="Save" value="Save Changes >>"id="Save" />
Ithen added the Add to Inventory and Cancel Editing buttons, indicatingwhich validation controls to disable in their respective NoFormValListattributes.
Using the Custom Control on the ServerThelast thing to look at is the .vb codebehind page, which handles all ofthe processing on the server side. One of the first things to do onthis page is include a reference to my new control. I did this with anormal Imports statement at the top of the page:
Imports MyApp.NoFormValControls
Within the class definition of the page itself, I hooked the pagecontrols into local controls, making sure I declared the buttons with atype of NoFormValButton.
Be sure to note the requirement of the Handles
ControlName.
Eventdirective at the end of each of the event declarations. If you omitthis directive, your procedures won‘t fire. For example, the Clickevent of the Add button should be declared:
Protected Sub Add_Click(ByVal Sender as object, _ByVal e as Eventargs) Handles Add.Click
Lastly,in each of the Click events for the buttons is a call to theDisableServerSideValidation method for the button. This disables theserver-side validation of the list of controls I defined back on the.aspx page. The call for the Save Changes button is simply:
Save.DisableServerSideValidation()
AfterI hooked in the other Click events, I was finished. When I tied thisall together, I got a page that allows you to have invalid input in theAdd to Inventory part, but still lets you cancel editing and savechanges. Also, it allows you to have invalid input in the EditInventory part, while leaving Cancel Editing and Add to Inventoryworking. As an added benefit (or perhaps the best benefit), you nowhave a custom server control that you can reuse in many otherapplications.
ConclusionFormvalidation controls are just one of the many new tools available todevelopers for performing the kinds of tasks that are frequentlyrequired of Web sites. If these tools aren‘t exactly what you need forall your scenarios, you can take advantage of the features of ASP.NETand Visual Basic .NET to override and extend these controls to makethem fit your particular needs.